An In-Depth Look at tqdm 4.65.0: A Python Library for Progress Bars in IT Automation
Explore the latest features of tqdm 4.65.0, a powerful Python library for progress bars. Learn how to use it for file transfers, data processing, and network requests in IT automation, with practical examples and customization tips.
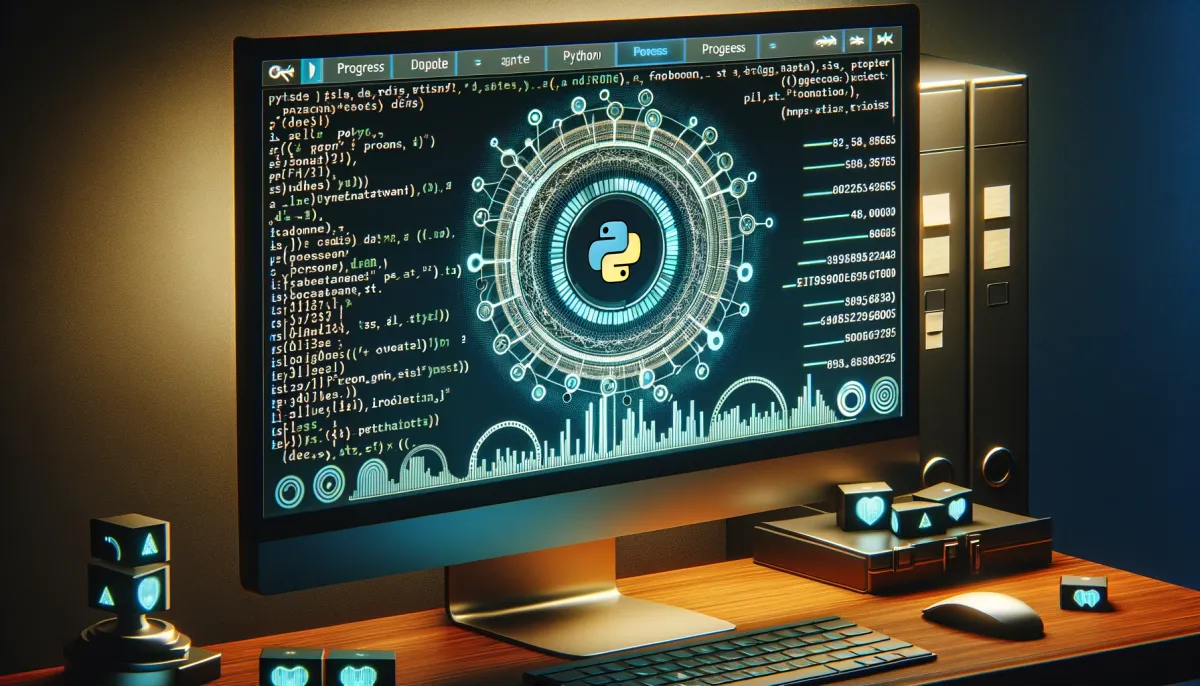
Introduction
Progress bars are a common and valuable tool in programming, particularly in scenarios where long-running tasks are involved. They provide visual feedback to users, indicating the progress of an operation and making the wait time more bearable. One of the most popular libraries for implementing progress bars in Python is tqdm
. As of this writing, the latest version is 4.66.4, but we will be using 4.65.0. This article will explore the features, enhancements, and applications of tqdm
4.65.0, with a focus on its use in IT automation.
What is tqdm
?
tqdm
(which means "progress" in Arabic) is a Python library that provides fast, extensible progress bars for loops and other iterable constructs. It is highly customizable and integrates well with various Python libraries, making it a go-to choice for developers needing progress visualization.
Key Features of tqdm
4.65.0
- Ease of Use:
tqdm
is designed to be user-friendly. A simple function call can wrap around an iterable to provide a progress bar. - Customizable: Users can customize the progress bar's appearance and behavior to fit their needs.
- Compatibility: It integrates seamlessly with other Python libraries such as
pandas
,requests
, andasyncio
. - Performance:
tqdm
is lightweight and does not significantly impact the performance of the wrapped code. - Rich Functionality: It supports nested progress bars, manual updates, and more.
Installation
To install tqdm
4.65.0, use pip:
pip install tqdm==4.65.0
Basic Usage
The simplest way to use tqdm
is to wrap it around an iterable. Here’s a basic example:
from tqdm import tqdm
import time
for i in tqdm(range(100)):
time.sleep(0.1) # Simulate some work
This code will display a progress bar that updates as the loop progresses.
Advanced Usage
Customizing the Progress Bar
You can customize the appearance and behavior of the progress bar using various parameters:
for i in tqdm(range(100), desc="Processing", ncols=80, ascii=True, unit="item"):
time.sleep(0.1)
desc
: A short description that will be displayed before the progress bar.ncols
: The width of the progress bar.ascii
: If set toTrue
, the bar will use ASCII characters.unit
: The unit of measurement for each iteration.
Progress Bars with pandas
tqdm
integrates well with pandas
, allowing you to visualize the progress of operations on DataFrames:
import pandas as pd
from tqdm import tqdm
tqdm.pandas(desc="Processing DataFrame")
df = pd.DataFrame({"a": range(100)})
df["b"] = df["a"].progress_apply(lambda x: x * 2)
Nested Progress Bars
tqdm
supports nested progress bars, which are useful for tracking progress in nested loops:
for i in tqdm(range(10), desc="Outer Loop"):
for j in tqdm(range(100), desc="Inner Loop", leave=False):
time.sleep(0.01)
The leave=False
parameter ensures that the inner loop’s progress bar disappears after completion, keeping the output clean.
Asynchronous Operations
tqdm
can be used with asynchronous operations, such as those involving asyncio
:
import asyncio
from tqdm.asyncio import tqdm
async def worker():
for i in tqdm(range(100), desc="Async Task"):
await asyncio.sleep(0.1)
asyncio.run(worker())
Real-World Applications in IT Automation
File Transfers
In IT automation, transferring files is a common task. tqdm
can be used to provide progress feedback during file transfers:
import os
import shutil
from tqdm import tqdm
src = "large_file.zip"
dst = "copy_large_file.zip"
total_size = os.path.getsize(src)
with open(src, 'rb') as fsrc, open(dst, 'wb') as fdst:
with tqdm(total=total_size, desc="Copying File", unit='B', unit_scale=True) as pbar:
while True:
buf = fsrc.read(1024)
if not buf:
break
fdst.write(buf)
pbar.update(len(buf))
Data Processing
Processing large datasets is another area where progress bars are invaluable. Whether it's parsing logs, transforming data, or performing calculations, tqdm
can help track the progress:
def process_data(item):
# Simulate data processing
time.sleep(0.01)
return item * 2
data = range(1000)
processed_data = [process_data(item) for item in tqdm(data, desc="Processing Data")]
Network Requests
Automated scripts often need to make multiple network requests. tqdm
can be integrated with requests
to monitor the progress of these requests:
import requests
from tqdm import tqdm
urls = ["http://example.com"] * 100
for url in tqdm(urls, desc="Fetching URLs"):
response = requests.get(url)
# Process the response
Enhancements in Version 4.65.0
The 4.65.0 release of tqdm
includes several improvements and bug fixes:
- Performance Enhancements: Optimizations for handling large iterables.
- Improved Compatibility: Better integration with modern libraries and frameworks.
- New Features: Additional parameters and methods for more granular control over progress bars.
- Bug Fixes: Various bug fixes to improve stability and reliability.
Conclusion
tqdm
4.65.0 is a powerful and flexible library for adding progress bars to Python applications. Its ease of use, customization options, and compatibility with other libraries make it an essential tool for developers, particularly in the field of IT automation. Whether you’re transferring files, processing data, or making network requests, tqdm
can help you provide better feedback to users and monitor the progress of your tasks effectively.
By leveraging the latest features and enhancements in version 4.65.0, you can ensure your automation scripts are both user-friendly and efficient.